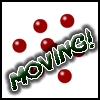
Good Morning!
It took a while to write that. Why the rum? Long story as a link: I saw that and liked it. Copy, paste, trace out all compile fails and rewrite and present!
The (re-coded) code:
var theScene:MovieClip = new MovieClip(); theScene.x = stage.stageWidth*0.5; theScene.y = stage.stageHeight*0.5; addChild( theScene ); var focalLength:Number = 300; function make3DPoint ( x, y, z) { var point = new Object(); point.x = x; point.y = y; point.z = z; return point; }; function make2DPoint (x,y, depth, scaleFactor) { var point = new Object(); point.x = x; point.y = y; point.depth = depth; point.scaleFactor = scaleFactor; return point; }; function Transform3DPointsTo2DPoints (points, axisRotations) { var TransformedPointsArray = []; var sx = Math.sin(axisRotations.x); var cx = Math.cos(axisRotations.x); var sy = Math.sin(axisRotations.y); var cy = Math.cos(axisRotations.y); var sz = Math.sin(axisRotations.z); var cz = Math.cos(axisRotations.z); var x,y,z, xy,xz, yx,yz, zx,zy, scaleFactor; var i = points.length; while (i--){ x = points[i].x; y = points[i].y; z = points[i].z; // rotation around x xy = cx*y - sx*z; xz = sx*y + cx*z; // rotation around y yz = cy*xz - sy*x; yx = sy*xz + cy*x; // rotation around z zx = cz*yx - sz*xy; zy = sz*yx + cz*xy; scaleFactor = focalLength/(focalLength + yz); x = zx*scaleFactor; y = zy*scaleFactor; z = yz; TransformedPointsArray[i] = make2DPoint(x, y, -z, scaleFactor); } return TransformedPointsArray; }; var pointsArray:Array = [ make3DPoint(-50,-50,-50), make3DPoint(50,-50,-50), make3DPoint(50,-50,50), make3DPoint(-50,-50,50), make3DPoint(-50,50,-50), make3DPoint(50,50,-50), make3DPoint(50,50,50), make3DPoint(-50,50,50) ]; var ballArray:Array = new Array(); for ( var i:int = 0; i < pointsArray.length; i++){ var ball:MovieClip = new MovieClip(); var colors = [ 0xffffff, 0x990000 ]; var fillType:String = GradientType.RADIAL; var alphas:Array = [1, 1]; var ratios:Array = [0x00, 0xff]; var matr:Matrix = new Matrix(); matr.createGradientBox(-40*0.4, -40*0.4, 0, 0, 0); var spreadMethod:String = SpreadMethod.PAD; var interpolationMethod:String = InterpolationMethod.RGB; var focal:Number = -0.1; with(ball.graphics) { beginGradientFill( fillType, colors, alphas, ratios, matr, spreadMethod, interpolationMethod, focal ); drawCircle(0,0,20); endFill(); } theScene.addChild(ball); ballArray.push(ball); } var cubeAxisRotations = make3DPoint(0,0,0); theScene.addEventListener( Event.ENTER_FRAME, enterFrameHandler ); function enterFrameHandler( event:Event ) { cubeAxisRotations.y -= 5*Math.PI/180; cubeAxisRotations.x += 5*Math.PI/180; var screenPoints = Transform3DPointsTo2DPoints(pointsArray, cubeAxisRotations); for (i=0; i < pointsArray.length; i++){ var currBall = ballArray[i]; currBall.x = screenPoints[i].x; currBall.y = screenPoints[i].y; currBall.xscale = currBall.yscale = 100 * screenPoints[i].scaleRatio; //currBall.swapDepths(screenPoints[i].depth); } }
Just don't ask. Yo... -ho!
EDIT: The spam comments, I forgot them. I don't like them. Like: I DON'T LIKE THEM!
EDIT_2: And: Creating the thumbnail was time consuming. More than writing...
EDIT_3: I forgot to include the depths.
function enterFrameHandler( event:Event ) { cubeAxisRotations.y -= 2*Math.PI/180; cubeAxisRotations.x += 2*Math.PI/180; var screenPoints = Transform3DPointsTo2DPoints(pointsArray, cubeAxisRotations); screenPoints.sortOn("depth"); for (i=0; i < screenPoints.length; i++){ var currBall = ballArray[i]; currBall.x = screenPoints[i].x; currBall.y = screenPoints[i].y; currBall.xscale = currBall.yscale = 100 * screenPoints[i].scaleRatio; theScene.addChild(currBall); } }